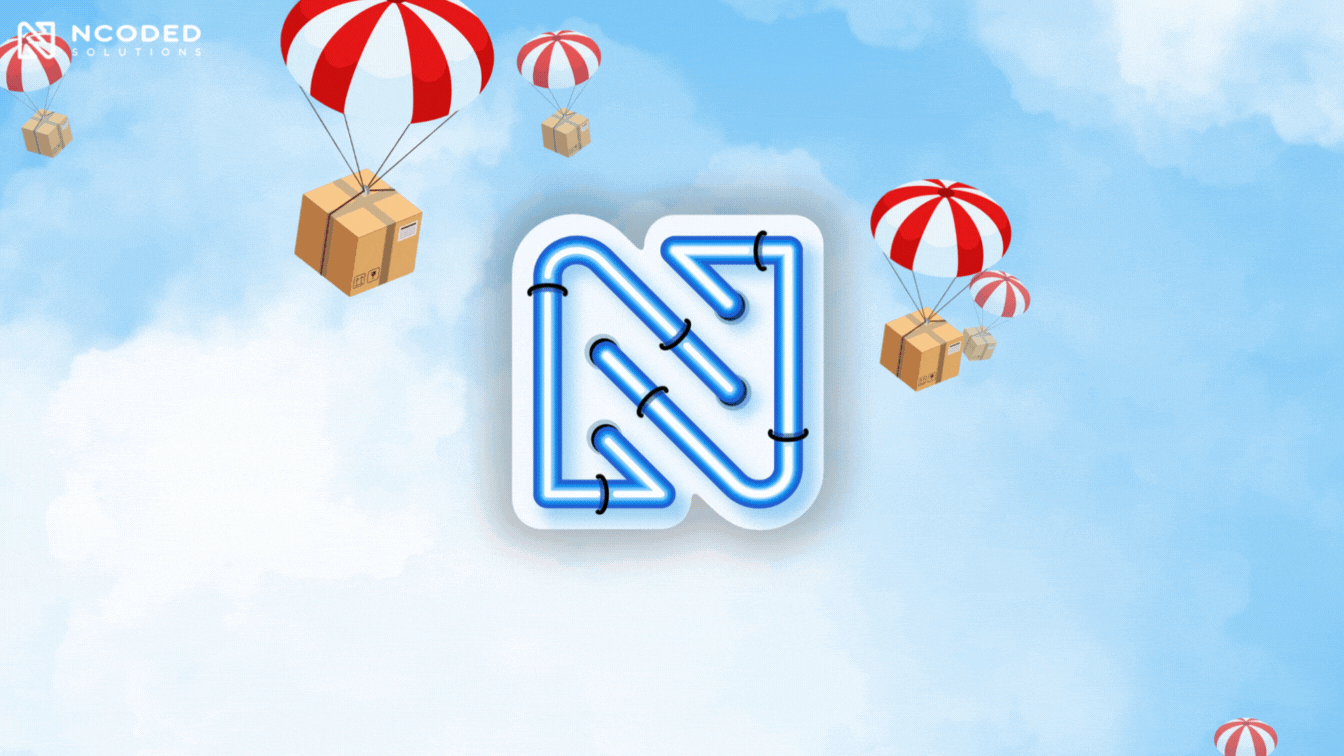
See all articles
May 29, 2024
Remember when deploying code used to be such a headache? Endless manual steps, unexpected errors and long downtimes made it a real pain for developers. Without an efficient workflow, too much time gets spent on setup instead of coding…
Thankfully, those days are behind us. Today, we have and , tools that make the deployment process much easier 😄
In this blog post, we'll explore how Vercel, combined with GitHub Actions, can automate your CI/CD pipeline, make the whole process smoother and take the stress out of deployments – except on Fridays.😅
At Ncoded Solutions, we've adopted this approach to keep our deployments as hassle-free as possible. We'll walk you through a simple tutorial for automating your deployment process, so keep scrolling.
First things first, what is Vercel?
No judgement here, but if you haven't heard of it yet, that's quite surprising… 🧐
Vercel is a big deal in the web development world because it makes deploying and managing web applications super easy. It’s a cloud based system that’s widely used by developers due to its seamless integration with popular frameworks and libraries like Next.js, React,** Angular** and Vue.js, providing a powerful and scalable environment for modern web projects. Actually, Vercel supports over 35 frameworks/libraries; you can find the full list .
The important thing is that your project needs to be hosted somewhere to be accessible to users on the web. In order to achieve that, it needs to be deployed to a server. Here are a few common options for doing this:
Each of these options requires considerable setup and configuration. This includes setting up authentication and networking to your local environment and possibly your backend server(s), caching your project globally for fast access, handling DNS to provide a user-friendly URL and more. While these tasks might seem overwhelming, they are crucial for a successful deployment.
Vercel, and its competition, are designed to simplify project deployment by automating many of these tasks, making the process as seamless as possible.
In the context of a CI/CD (Continuous Integration and Continuous Deployment), Vercel plays a key role in the deployment phase. It aims to make the software development process faster and more efficient.
Continuous Integration involves frequently merging code changes into a shared repository. Automated testing and building ensure that the application functions correctly before deployment. These tasks can be automated using GitHub Actions:
GitHub Actions can run a suite of tests on your application every time you push new code, ensuring that your changes don’t break existing functionality. This helps in identifying and fixing bugs early in the development cycle. GitHub Actions can also handle the build process, compiling your code into a production-ready format.
By automating these steps, CI reduces the risk of integration issues and improves the overall quality of the software.
Once your application has successfully passed all tests in the CI phase, it’s time for Continuous Deployment, where Vercel takes the spotlight:
Vercel automatically deploys the latest version of your application as soon as it passes the CI tests. This ensures that your production environment is always up-to-date with the latest code changes. By integrating Vercel with GitHub Actions, any code merged into your main branch can trigger an automatic deployment, minimizing manual intervention and speeding up the release cycle.
Simply put: CI is a set of practices performed as developers are writing code, while CD is a set of practices performed after the code is completed.
GitHub Actions is a tool integrated with GitHub that allows you to build, test and deploy your code with ease. By setting up workflows, you can define a series of actions that run automatically in response to events in your repository, such as code pushes or pull requests.
GitHub workflows are part of the GitHub Actions and they’re automated processes that you can set up directly in your GitHub repository. Workflows allow you to automate a wide range of tasks, from running tests to deploying applications and sending notifications. They’re defined with YAML files in the .github/workflows folder of your project. Key components of workflows are: triggers, jobs and steps.
Okay, now you’re ready to start our tutorial 👇🏼
First of all, if you don’t have a project yet, you should create one as soon as possible. After your project is created, make your first commit and push it in order to link the project with your GitHub repository.
In the root project folder, create a new folder named .github and a subfolder named workflows.
For each pipeline or a workflow you want to create, you’ll need a separate YAML file inside of the workflows folder.
In our case, we’ll make two: a production and a preview deployment pipeline, so let’s name the files accordingly: production.yaml and preview.yaml.
In your new yaml file .github/workflows/production.yaml, copy and paste the following code:
name: Vercel Production Deployment
env:
VERCEL_ORG_ID: ${{ secrets.VERCEL_ORG_ID }}
VERCEL_PROJECT_ID: ${{ secrets.VERCEL_PROJECT_ID }}
on:
push:
branches:
- main
jobs:
Deploy-Production:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Vercel CLI
run: npm install --global vercel@latest
- name: Pull Vercel Environment Information
run: vercel pull --yes --environment=production --token=${{ secrets.VERCEL_TOKEN }}
- name: Build Project Artifacts
run: vercel build --prod --token=${{ secrets.VERCEL_TOKEN }}
- name: Deploy Project Artifacts to Vercel
run: vercel deploy --prebuilt --prod --token=${{ secrets.VERCEL_TOKEN }}
For better understanding, we’ll explain each part of this file:
name: Vercel Production Deployment
env:
VERCEL_ORG_ID: ${{ secrets.VERCEL_ORG_ID }}
VERCEL_ORG_ID: ${{ secrets.VERCEL_ORG_ID }}
VERCEL_PROJECT_ID: ${{ secrets.VERCEL_PROJECT_ID }}
on:
push:
branches:
- main
For production, our production pipeline should start only when something is pushed to the main branch. Here, we specify every push into the repository, but then define a filter for certain branches.
jobs:
Deploy-Production:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Vercel CLI
run: npm install --global vercel@latest
- name: Pull Vercel Environment Information
run: vercel pull --yes --environment=production --token=${{ secrets.VERCEL_TOKEN }}
- name: Build Project Artifacts
run: vercel build --prod --token=${{ secrets.VERCEL_TOKEN }}
- name: Deploy Project Artifacts to Vercel
run: vercel deploy --prebuilt --prod --token=${{ secrets.VERCEL_TOKEN }}
This step deploys the prebuilt project artefacts to Vercel using the token stored in GitHub Secrets. The --prebuilt flag is passed since we did a building in the previous step.
That’s it, the Production pipeline is all set up! 🚀
For the Preview pipeline, the file is pretty similar with some simple changes:
name: Vercel Preview Deployment
env:
VERCEL_ORG_ID: ${{ secrets.VERCEL_ORG_ID }}
VERCEL_PROJECT_ID: ${{ secrets.VERCEL_PROJECT_ID }}
on:
push:
branches-ignore:
- main
jobs:
Deploy-Preview:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Vercel CLI
run: npm install --global vercel@latest
- name: Pull Vercel Environment Information
run: vercel pull --yes --environment=preview --token=${{ secrets.VERCEL_TOKEN }}
- name: Build Project Artifacts
run: vercel build --token=${{ secrets.VERCEL_TOKEN }}
- name: Deploy Project Artifacts to Vercel
run: vercel deploy --prebuilt --token=${{ secrets.VERCEL_TOKEN }}
After you commit and push the changes to GitHub repository, in Actions section you’ll see something like this:
The pipeline is running (because we did a push to the main branch), but it failed. Why? Because the variables that are needed in the pipeline are not present yet.
In order to get those variables, we’ll need to create a Vercel project.
Go to vercel.com and make a free account, if you don’t have one already. You can choose to authenticate either with a Git provider or by using an email.
Vercel CLI needs an API access token, so in addition to that we need an org ID and a project ID of the project.
Once you’re logged in, go to Account Settings > Tokens and create a new token.
Define a name, scope and an expiration date and then click on the Create button. Copy your token and go to your GitHub repository Settings and under Secrets and variables choose Actions. Create a new repository secret named VERCEL_TOKEN and paste your token in the Secret section.
Now we can create a Vercel project and we can do it using the Vercel CLI locally.
First, install the Vercel CLI:
npm i -g vercel
Then login into a Vercel account:
vercel login
Create a Vercel project using:
vercel link
This command will ask you a few questions:
Vercel recognizes the framework automatically so it suggests appropriate build and install commands for your project. You can modify these if you want, in this tutorial we won’t do it.
The result of the vercel link command is the .vercel subfolder in your project. It contains the project.json file with project and org IDs.
Copy those two values and make two new secrets in your GitHub repository. The first one should be called VERCEL_PROJECT_ID and the second one VERCEL_ORG_ID.
Our Vercel configuration is done. Now we can test out the pipelines!
Let’s first try out the Preview pipeline. If you remember it will trigger when we make a push on any brunch but the main. So, let’s create a new branch, make some changes and push them. In your GitHub repository in Actions section you’ll see the Preview pipeline running:
To test our Production pipeline we can simply merge our new pull request into the main branch:
With everything set up, Vercel will automatically detect your future commits and seamlessly deploy your project.
You can make your pipelines more complex by adding automated testing. Just add this peace of code into your yaml file, before the build step:
- name: Run the tests
run: npm run test
This way, every change gets a thorough check-up before going live, giving you peace of mind and extra confidence in your deployments.
Automatic deployment in Extreme Programming (XP) When talking about automated deployment, Extreme Programming (XP) is definitely something worth mentioning. It’s an agile software development methodology that focuses on frequent releases in short development cycles.
Automating your deployment process is incredibly helpful in XP because it makes these frequent deployments fast and reliable. With a good CI/CD pipeline and thorough tests, you can ensure that every change is tested and ready for production without manual intervention. This means the code is always in a deployable state, reducing errors, saving time and allowing your team to focus on creating high-quality code.
Moreover, automated deployment supports XP's emphasis on continuous feedback. By quickly deploying updates, you can gather user feedback sooner and iterate on your software more effectively.
By now, it should be obvious, but let’s make it clearer now:
Speed and efficiency – manual deployments are time-consuming and prone to errors, so automating this process allows you to have rapid, consistent deployments with a reduced time between writing code and getting it into production. This means quicker iteration, faster release cycles and the better response to customer feedback.
Consistency and reliability – with automatic deployments every deployment will follow the exact same steps, eliminating the factor of human errors that often accompany manual processes. So, automated deployments are consistent, repeatable and standardized.
Scalability – as your project grows, the complexity and frequency of deployments are likely to increase. Automated deployment processes scale effortlessly with your needs.
Productivity and better Developer experience – developers can focus more on what they do the best – writing code and less on the manual work – the deployment process. This improves productivity and fosters better collaboration among team members 🤝
Choosing the right platform for automated deployment is crucial to maximizing the benefits of the automation process. You probably noticed our enthusiasm for Vercel in this blog, well here’s why:
When it comes to CI/CD tools, developers have a variety of options to choose from. So, let’s compare Vercel with other popular CI/CD tools like Jenkins, CircleCI and Travis CI.
While Jenkins, CircleCI, and Travis CI each have their strengths, Vercel stands out for its simplicity, efficiency and seamless integration with modern web development frameworks.
At Ncoded Solutions, these advantages make Vercel our preferred choice for automating our CI/CD pipelines.
Now that you've seen how Vercel and GitHub Actions can revolutionize your deployment workflow, what are your thoughts?
How do you envision integrating CI/CD pipelines into your projects, and what challenges do you anticipate?
Share your experiences and ideas in the comments below! 🤝
You may also like
You need to find a dedicated software team? Here’s how to do it #16
Learn how to choose the right dedicated development team that fits your product, your goals, and your way of working.
July 10, 2025tips-and-tricks
Can AI code my app? #15
We asked AI to make a real application based on given requirements. The results? Far from a real product.
May 30, 2025tech